Assets文件介绍
assets文件夹里面的文件都是保持原始的文件格式,需要用AssetManager以字节流的形式读取文件。
1. 先在Activity里面调用getAssets() 来获取AssetManager引用。
2. 再用AssetManager的open(String fileName, int accessMode) 方法则指定读取的文件以及访问模式就能得到输入流InputStream。
3. 然后就是用已经open file 的inputStream读取文件,读取完成后记得inputStream.close() 。
4. 调用AssetManager.close() 关闭AssetManager。
封装类
代码遵循单例模式,例如:
import android.content.Context;import android.os.Environment;import android.os.Handler;import android.os.Looper;import android.os.Message;import java.io.File;import java.io.FileOutputStream;import java.io.InputStream;/** * Created by shenhua on 1/17/2017. * Email shenhuanet@126.com */public class FileUtils { private static FileUtils instance; private static final int SUCCESS = 1; private static final int FAILED = 0; private Context context; private FileOperateCallback callback; private volatile boolean isSuccess; private String errorStr; public static FileUtils getInstance(Context context) { if (instance == null) instance = new FileUtils(context); return instance; } private FileUtils(Context context) { this.context = context; } private Handler handler = new Handler(Looper.getMainLooper()) { @Override public void handleMessage(Message msg) { super.handleMessage(msg); if (callback != null) { if (msg.what == SUCCESS) { callback.onSuccess(); } if (msg.what == FAILED) { callback.onFailed(msg.obj.toString()); } } } }; public FileUtils copyAssetsToSD(final String srcPath, final String sdPath) { new Thread(new Runnable() { @Override public void run() { copyAssetsToDst(context, srcPath, sdPath); if (isSuccess) handler.obtainMessage(SUCCESS).sendToTarget(); else handler.obtainMessage(FAILED, errorStr).sendToTarget(); } }).start(); return this; } public void setFileOperateCallback(FileOperateCallback callback) { this.callback = callback; } private void copyAssetsToDst(Context context, String srcPath, String dstPath) { try { String fileNames[] = context.getAssets().list(srcPath); if (fileNames.length > 0) { File file = new File(Environment.getExternalStorageDirectory(), dstPath); if (!file.exists()) file.mkdirs(); for (String fileName : fileNames) { if (!srcPath.equals("")) { // assets 文件夹下的目录 copyAssetsToDst(context, srcPath + File.separator + fileName, dstPath + File.separator + fileName); } else { // assets 文件夹 copyAssetsToDst(context, fileName, dstPath + File.separator + fileName); } } } else { File outFile = new File(Environment.getExternalStorageDirectory(), dstPath); InputStream is = context.getAssets().open(srcPath); FileOutputStream fos = new FileOutputStream(outFile); byte[] buffer = new byte[1024]; int byteCount; while ((byteCount = is.read(buffer)) != -1) { fos.write(buffer, 0, byteCount); } fos.flush(); is.close(); fos.close(); } isSuccess = true; } catch (Exception e) { e.printStackTrace(); errorStr = e.getMessage(); isSuccess = false; } } public interface FileOperateCallback { void onSuccess(); void onFailed(String error); }}
调用代码
如果你需要将如图所示的apks下的文件复制到SD卡的app/apks目录下,则这样调用:
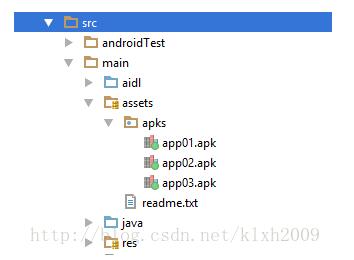
FileUtils.getInstance(Context context).copyAssetsToSD("apks","app/apks");
###如果你需要收到文件复制完成的时的回调,则使用如下代码:
FileUtils.getInstance(Context context).copyAssetsToSD("apks","app/apks").setFileOperateCallback(new FileUtils.FileOperateCallback() { @Override public void onSuccess() { // TODO: 文件复制成功时,主线程回调 } @Override public void onFailed(String error) { // TODO: 文件复制失败时,主线程回调 } });
代码说明
在上面代码中,通过单例模式传入一个context获得FileUtils实例,通过实例去调用copyAssetsToSD()方法,方法参数:
- String srcPath 传入assets文件夹下的某个文件夹名,如上述apks,可传入为空”“字符,则复制到SD后,默认将assets文件夹下所有文件复制;
- String sdPath 传入你希望将文件复制到的位置,如SD卡下的“abc”文件夹,则传入”abc”
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持VEVB武林网。
注:相关教程知识阅读请移步到Android开发频道。